邮件发送
创建一个springboot项目
用IDEA创建springboot项目。
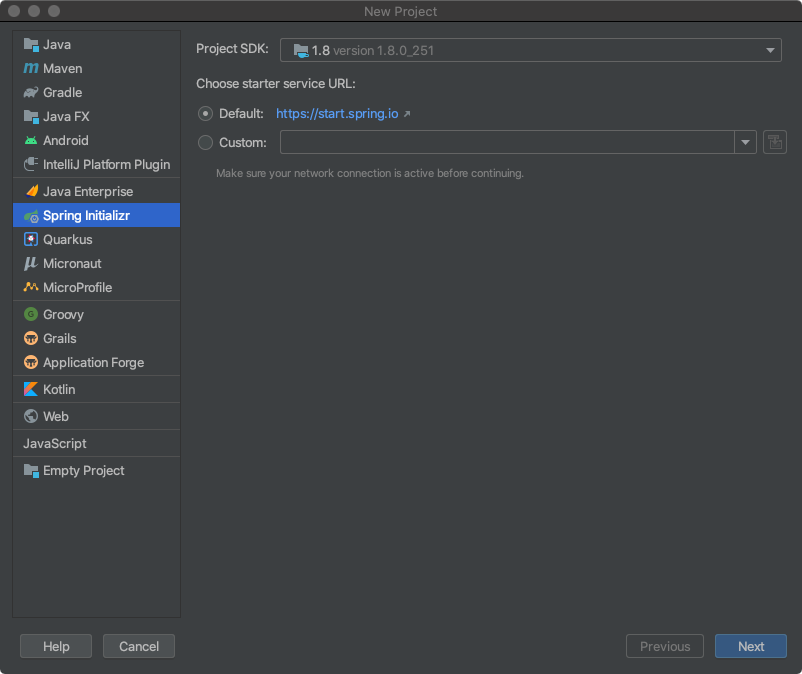
之后可以一直next,依赖之后说。
添加依赖
打开pom.xml,添加如下依赖:
1 2 3 4
| <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-mail</artifactId> </dependency>
|
修改配置文件
打开application.properties,添加如下内容:
1 2 3 4 5 6 7
| spring.mail.username=xxxxxx@163.com spring.mail.password=网易账号授权码 spring.mail.host=smtp.163.com
##spring.mail.properties.mail.smtp.ssl.enable=true
|
关于授权码,注意不是你的邮箱密码,位置如下所示。
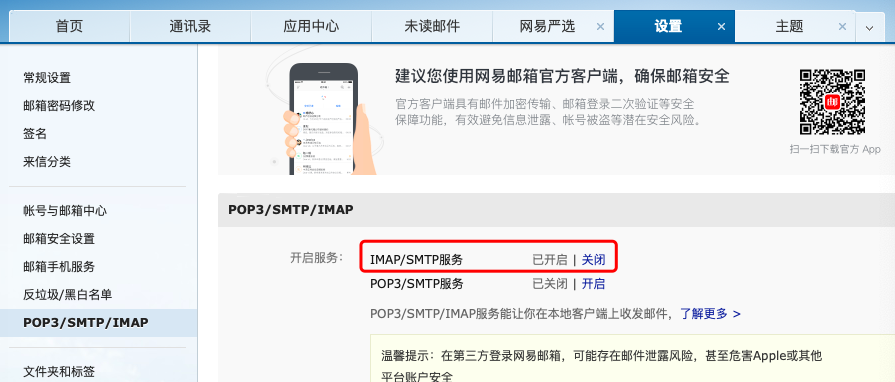
只要选择开启之后发个短信即可获取。
测试类
首先在测试类要注入JavaMailSenderImpl
1 2
| @Autowired private JavaMailSenderImpl mailSender;
|
运行测试类进行邮件发送
1 2 3 4 5 6 7 8 9
| @Test void contextLoads() { SimpleMailMessage simpleMailMessage = new SimpleMailMessage(); simpleMailMessage.setSubject("主题"); simpleMailMessage.setText("内容"); simpleMailMessage.setFrom("xxxxxx@163.com"); simpleMailMessage.setTo("xxxxxx@163.com"); mailSender.send(simpleMailMessage); }
|
或许你可能一次成功,或许你失败了,失败的原因80%都是这个。
1
| org.springframework.mail.MailAuthenticationException: Authentication failed; nested exception is javax.mail.AuthenticationFailedException: 535 Error: xxxxxxx: http:
|
说明你的授权码不对,那就再试试吧。
到这一步,你已经实现了一封由springboot发送的邮件。
实现异步
需要提前添加web依赖。
修改启动类
添加@EnableAsync注解,开启异步操作
1 2 3 4 5 6 7
| @EnableAsync @SpringBootApplication public class DemoApplication { public static void main(String[] args) { SpringApplication.run(DemoApplication.class, args); } }
|
创建Controller层和Service层
Service层
创建一个AsyncService类:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| package Service;
import org.springframework.scheduling.annotation.Async; import org.springframework.stereotype.Service;
@Service public class AsyncService { @Async public void async() { try { Thread.sleep(10000); } catch (InterruptedException e) { e.printStackTrace(); } System.out.println("数据正在处理"); } }
|
Controller层 创建一个AsyncController类: 页面访问,当我们请求服务器时,遇到线程方法会停止10秒。
随便玩玩,不要求获取方式了。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| package Controller;
import Service.AsyncService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController;
@RestController public class AsyncController { @Autowired AsyncService asyncService;
@RequestMapping("/async") public String async() { asyncService.async(); return "yes"; } }
|
添加@Async注解,实现异步操作
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| package Controller;
import Service.AsyncService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.scheduling.annotation.Async; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController;
@RestController public class AsyncController { @Autowired AsyncService asyncService;
@Async @RequestMapping("/async") public String async() { asyncService.async(); return "yes"; } }
|
本文作者:jujimeizuo
本文地址: https://blog.jujimeizuo.cn/2021/02/17/springboot-realize-mail-sending-asynchronous-function/
本博客所有文章除特别声明外,均采用 CC BY-SA 3.0 协议。转载请注明出处!